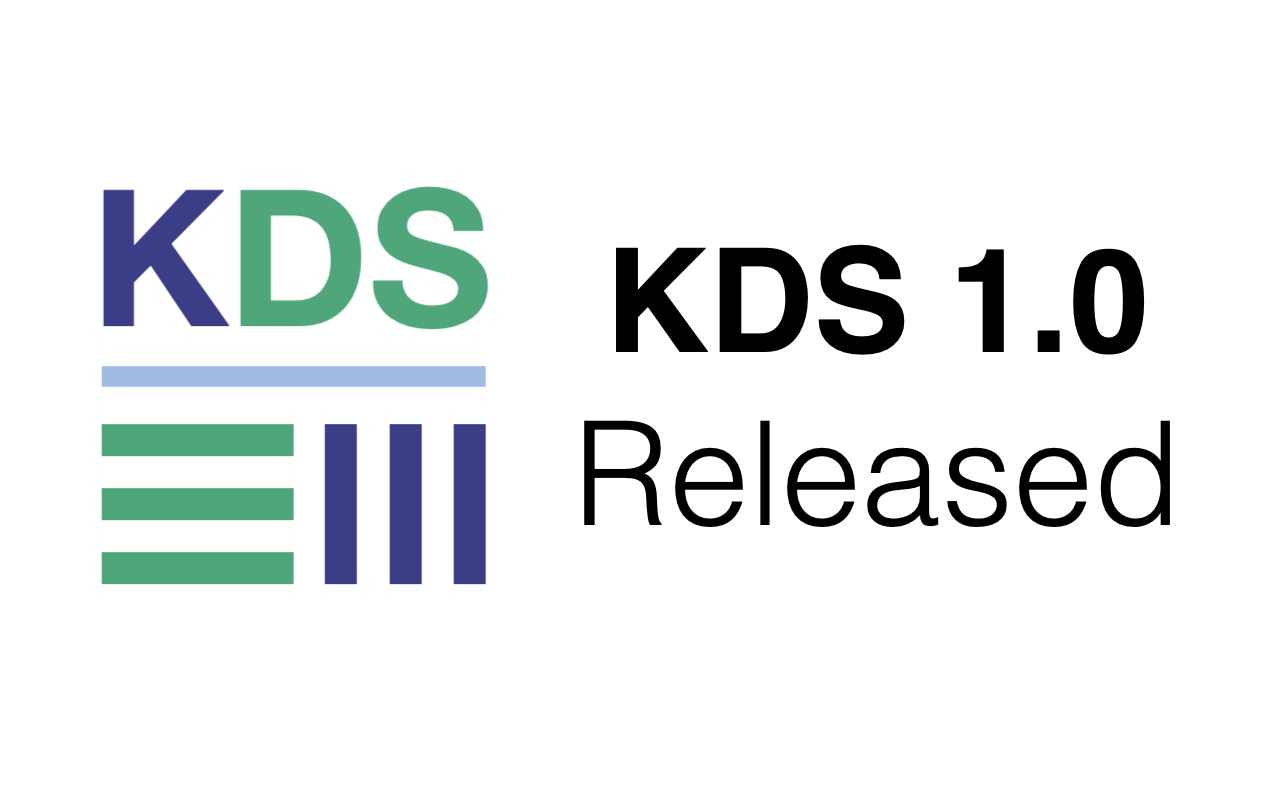
Released Kds 1.0: A Data Structure library for Multiplatform Kotlin 1.3
KORLIBS , RELEASE , KORGE
December 16, 2018
Last month I released Klock
. Now it is the turn of Kds to be released.
Kds is a Data Structure library for Multiplatform Kotlin 1.3.
It is designed to provide specialized collections that prevent boxing like IntArrayList or IntIntMap, and to provide some collections and utilities that are missing from the Kotlin's standard library at the moment.
Kds is part of my set of libraries for Kotlin called Korlibs. And along Klock it is one of the libraries from the first layer of the stack.
- Github: https://github.com/korlibs/kds
- Documentation: https://korlibs.soywiz.com/kds/
A few samples:
// Case Insensitive Map
val map = mapOf("hELLo" to 1, "World" to 2).toCaseInsensitiveMap()
println(map["hello"])
// BitSet
val array = BitSet(100) // Stores 100 bits
array[99] = true
// TypedArrayList
val v20 = intArrayListOf(10, 20).getCyclic(-1)
// Deque
val deque = IntDeque().apply {
addFirst(n)
removeFirst()
addLast(n)
}
// CacheMap
val cache = CacheMap<String, Int>(maxSize = 2).apply {
this["a"] = 1
this["b"] = 2
this["c"] = 3
assertEquals("{b=2, c=3}", this.toString())
}
// IntIntMap
val m = IntIntMap().apply {
this[0] = 98
}
// Pool
val pool = Pool { Demo() }
pool.alloc { demo ->
println("Temporarilly allocated $demo")
}
// Priority Queue
val pq = IntPriorityQueue()
pq.add(10)
pq.add(5)
pq.add(15)
assertEquals(5, pq.removeHead())
// Extra Properties
class Demo : Extra by Extra.Mixin() { val default = 9 }
var Demo.demo by Extra.Property { 0 }
var Demo.demo2 by Extra.PropertyThis<Demo, Int> { default }
val demo = Demo()
assertEquals(0, demo.demo)
assertEquals(9, demo.demo2)
demo.demo = 7
assertEquals(7, demo.demo)
assertEquals("{demo=7, demo2=9}", demo.extra.toString())
// mapWhile
val iterator = listOf(1, 2, 3).iterator()
assertEquals(listOf(1, 2, 3), mapWhile({ iterator.hasNext() }) { iterator.next()})
// And much more!