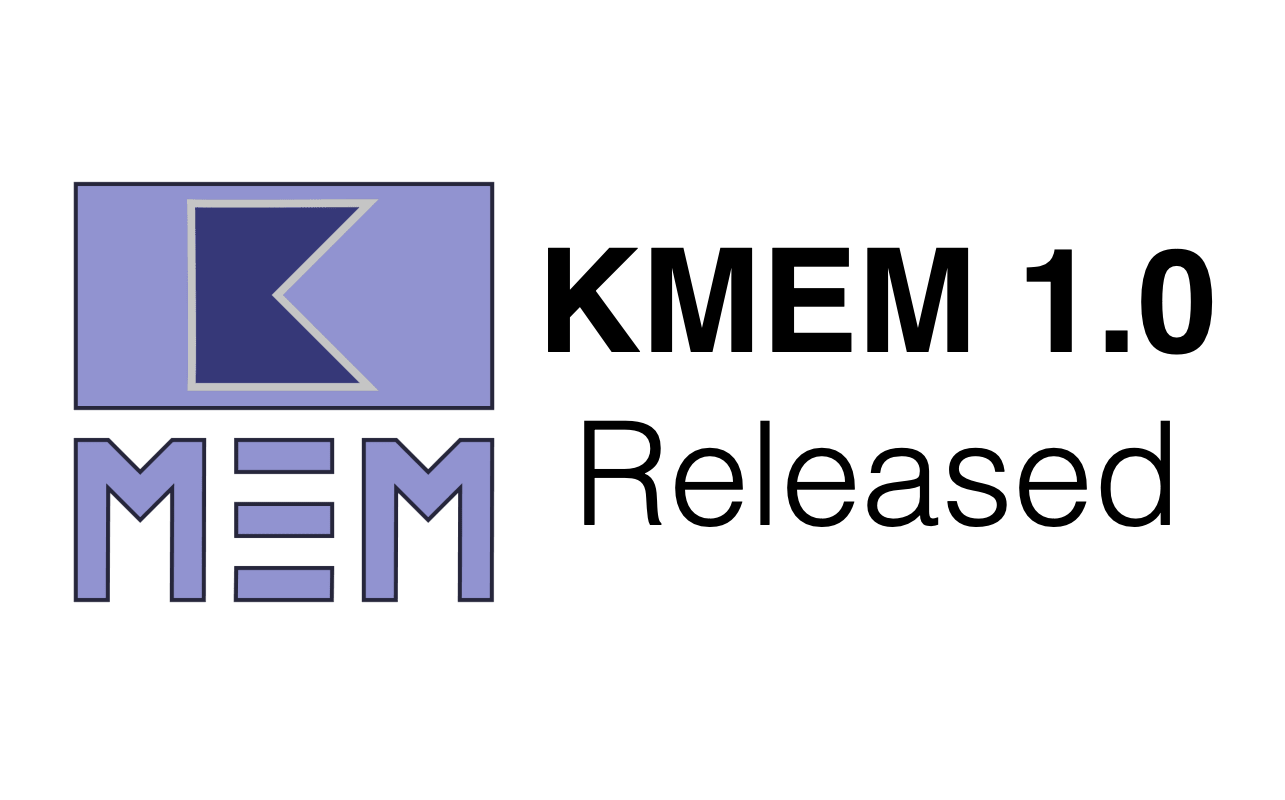
Released Kmem 1.0: Bit, Array and Fast Memory utilities for Multiplatform Kotlin 1.3
KORLIBS , RELEASE , KORGE
December 19, 2018
A few days ago I released Kds
, and last month I released Klock
. Now it is the turn of Kmem to be released.
Kmem is a Bit, Array and Fast Memory utilities library for Multiplatform Kotlin 1.3.
This library provides extension methods and properties useful for memory handling, and bit manipulation, as well as array and buffer similar to JS typed arrays.
Kmem is part of my set of libraries for Kotlin called Korlibs. And along Kds and Klock it is one of the libraries from the first layer of the stack.
- Github:
https://github.com/korlibs/kmem
- Documentation:
https://korlibs.soywiz.com/kmem/
A few samples:
// Array copying
val array = arrayOf("a", "b", "c", null, null)
arraycopy(array, 0, array, 1, 4)
// Array filling
val array = intArrayOf(1, 1, 1, 1, 1)
array.fill(2)
assertEquals(intArrayOf(2, 2, 2, 2, 2).toList(), array.toList())
// CLZ32
assertEquals(1, (0b11111111111111111111111111111110).toInt().countTrailingZeros())
// Byte Array building
val byteArray = buildByteArray {
append(1)
append(2)
append(byteArrayOf(3, 4, 5))
s32LE(6)
}
// Byte Array reading
val byteArray = buildByteArray { f32BE(1f).f32LE(2f) }
byteArray.read {
assertEquals(1f, f32BE())
assertEquals(2f, f32LE())
}
// Float16
assertEquals(+1.0, Float16.fromBits(0x3c00).toDouble())
// Power of Two
assertEquals(16, 10.nextPowerOfTwo)
assertEquals(16, 17.prevPowerOfTwo)
assertEquals(true, 1024.isPowerOfTwo)
// Aligned (multiple of)
assertEquals(false, 77.isAlignedTo(10))
assertEquals(70, 77.prevAlignedTo(10))
assertEquals(80, 77.nextAlignedTo(10))
// ByteArray indexed typed reading
val v = byteArray.readS32LE(10)
// Buffers
val mem = FBuffer.alloc(10)
for (n in 0 until 8) mem[n] = n
assertEquals(0x03020100, mem.getAlignedInt32(0))
assertEquals(0x07060504, mem.getAlignedInt32(1))