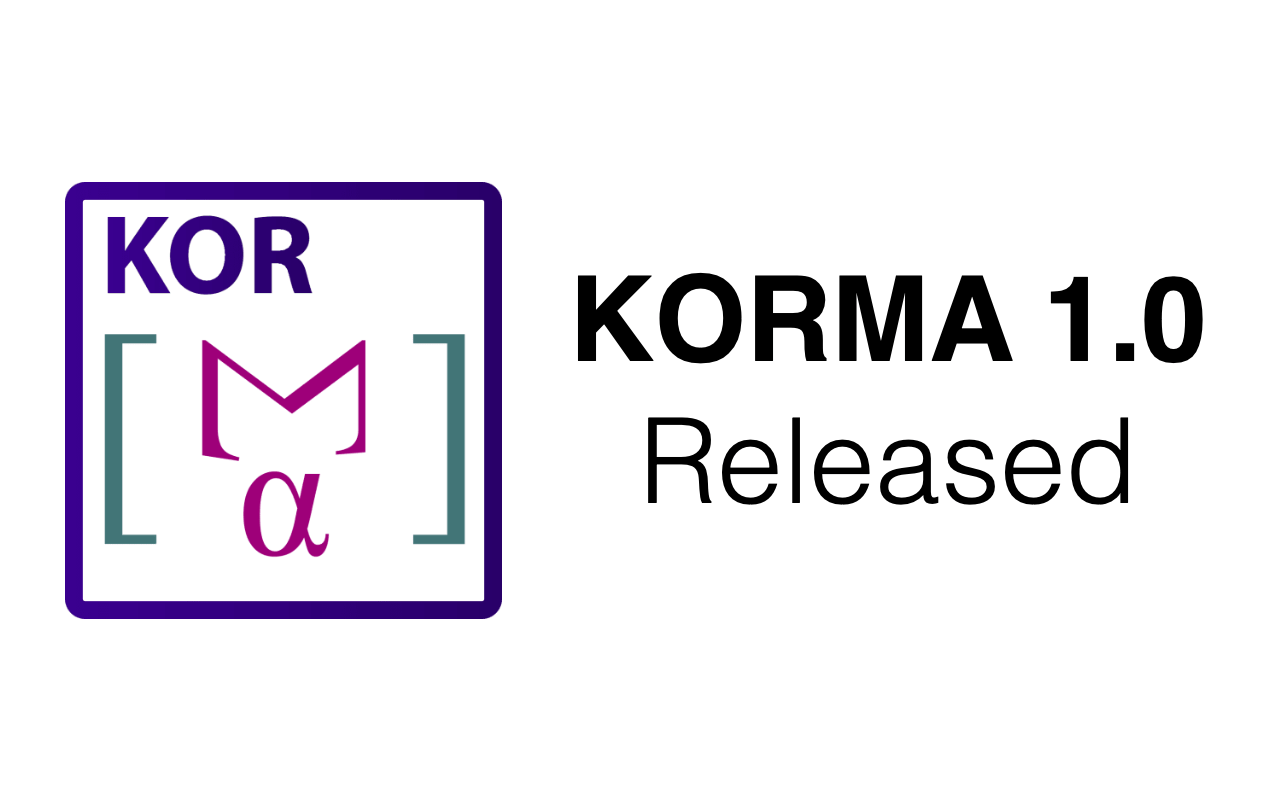
Released Korma 1.0: A Math library focused on geometry for Multiplatform Kotlin 1.3
KORLIBS , RELEASE , KORGE
December 29, 2018
After releasing the first layer of Korlibs (including Klock
, Kds
and Kmem
) I have started to polish the second layer of the stack (Korma
and Korio
). And I have just finished the first version of Korma.
Korma is a Mathematical Library mostly focused on geometry for Multiplatform Kotlin 1.3.
It includes structures for Points and Matrices (2D and 3D), Typed Angles, Rectangles, BoundsBuilder, Anchors, Vector graphics with Bezier curves support and Context2D-like API for building vectors, Interpolation facilities, Easing, Triangulation, BinPacking and Path Finding in Bidimensional arrays and Triangulated Spatial Meshes.
- Github: https://github.com/korlibs/korma
- Documentation: https://korlibs.soywiz.com/korma/
A few samples:
val vector = VectorPath {
// Here we can use moveTo, lineTo, quadTo, cubicTo, circle, ellipse, arc...
rect(0, 0, 100, 100)
rect(300, 0, 100, 100)
}.triangulate().toString()
// "[Triangle((0, 100), (100, 0), (100, 100)), Triangle((0, 100), (0, 0), (100, 0))], [Triangle((300, 100), (400, 0), (400, 100)), Triangle((300, 100), (300, 0), (400, 0))](/triangle((0,-100),-(100,-0),-(100,-100)),-triangle((0,-100),-(0,-0),-(100,-0))],-[triangle((300,-100),-(400,-0),-(400,-100)),-triangle((300,-100),-(300,-0),-(400,-0))/)"
// Angles
val angle = 90.degrees
val angleInRadians = angle.radians
// Matrices
val a = Matrix(2, 1, 1, 2, 10, 10)
val b = a.inverted()
assertEquals(identity, a * b)
// Rectangle + ScaleMode + Anchor
assertEquals(Rectangle(0, -150, 600, 600), Size(100, 100).applyScaleMode(Rectangle(0, 0, 600, 300), ScaleMode.COVER, Anchor.MIDDLE_CENTER))
// PathFinding (Matrix)
val points = AStar.find(
board = Array2("""
.#....
.#.##.
.#.#..
...#..
""") { c, x, y -> c == '#' },
x0 = 0,
y0 = 0,
x1 = 4,
y1 = 2,
findClosest = false
)
println(points)
// [(0, 0), (0, 1), (0, 2), (0, 3), (1, 3), (2, 3), (2, 2), (2, 1), (2, 0), (3, 0), (4, 0), (5, 0), (5, 1), (5, 2), (4, 2)]
// PathFinding (Shape)
assertEquals(
"[(10, 10), (100, 50), (120, 52)]",
(Rectangle(0, 0, 100, 100).toShape() + Rectangle(100, 50, 50, 50).toShape()).pathFind(
IPoint(10, 10),
IPoint(120, 52)
).toString()
)